 |
 |
|
How To Use Regular Java Classes With JSP
In
this topic, you'll learn how to use regular Java classes to do the
processing that a JSP requires. In particular, you'll learn how to use two
classes named User and UserIO to do the processing for the JSP of the
Email List application.
The
code for the User and UserIO classes
Figure 4-9 presents the code for a business class named User and an I/O
class named UserIO. The package statement at the start of each class
indicates where each class is stored. Here, the User class is stored in
the business directory because it defines a business object while the
UserIO class is stored in the data directory because it provides the data
access for the application.
Figure 4-9: The code for the User and UserIO classes
The code for the User class
package business;
public class User{
private String firstName;
private String lastName;
private String emailAddress;
public User(){}
public User(String first, String last, String email){
firstName = first;
lastName = last;
emailAddress = email;
}
public void setFirstName(String f){
firstName = f;
}
public String getFirstName(){ return firstName; }
public void setLastName(String l){
lastName = l;
}
public String getLastName(){ return lastName; }
public void setEmailAddress(String e){
emailAddress = e;
}
public String getEmailAddress(){ return emailAddress; }
}
The code for the UserIO class
package data;
import java.io.*;
import business.User;
public class UserIO{
public synchronized static void addRecord
(User user, String filename)
throws IOException{
PrintWriter out = new PrintWriter(
new FileWriter(filename, true));
out.println(user.getEmailAddress()+ "|"
+ user.getFirstName() + "|"
+ user.getLastName());
out.close();
}
}
Note
The synchronized keyword in the declaration for the addRecord method of
the UserIO class prevents two users of the JSP from using that method at
the same time.
The
User class defines a user of the application. This class contains three
instance variables: firstName, lastName, and emailAddress. It includes a
constructor that accepts three values for these instance variables. And it
includes get and set methods for each instance variable.
In contrast, the UserIO class contains one static method named addRecord
that writes the values stored in a User object to a text file. This method
accepts two parameters: a User object and a string that provides the path
for the file. If this file exists, the method will add the user data to
the end of it. If the file doesn't exist, the method will create it and
add the data at the beginning of the file.
If you've read the first six chapters of Murach's
Beginning Java 2, you should understand the code for the User class.
And if you've read chapters 16 and 17, you should understand the code in
the UserIO class. The one exception is the use of the synchronized keyword
in the addRecord method declaration. But this keyword just prevents two
users from writing to the file at the same time, which could lead to an
error.
Where and how to save and compile regular Java classes
If you're using Tomcat 4.0, figure 4-10 shows where and how to save your
compiled Java classes (the .class files) so Tomcat can access them.
Usually, you'll save your source code (the .java files) in the same
directory, but that's not required.
Figure 4-10: Where and how to save and compile regular Java classes
Where the User class is saved
c:tomcatwebappsmurachWEB-INFclassesbusiness
Where the UserIO class is saved
c:tomcatwebappsmurachWEB-INFclassesdata
Other places to save your Java classes
c:tomcatwebappsyourDocumentRootWEB-INFclasses
c:tomcatwebappsyourDocumentRootWEB-INFclassespackageName
c:tomcatwebappsROOTWEB-INFclasses
c:tomcatwebappsROOTWEB-INFclassespackageName
The DOS prompt window for compiling the User class
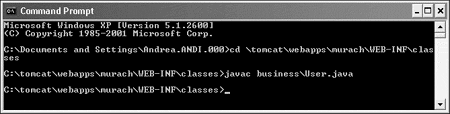
Description
-
Although
you can save the source code (the .java files) in any directory, you
must save the class files (the .class files) in the WEB-INFclasses
directory or one of its subdirectories. This can be subordinate to the
ROOT directory or your own document root directory.
-
To
compile a class, you can use TextPad's Compile Java command, your
IDE's compile command, or the javac command from the DOS prompt
window.
-
If
you have trouble compiling a class, make sure your system is
configured correctly as described in appendix A.
The two paths shown at the top of this figure show where the User and
UserIO classes that come with this book are saved. After that, the figure
presents the syntax for other paths that can be used to store Java
classes. If you review these paths, you'll see that each one places the
Java classes in a subdirectory of the WEB-INFclasses directory.
Since the User class contains a package statement that corresponds to the
business directory, it must be located in the WEB-INFclassesbusiness
directory. In contrast, if the package statement specified "murach.email",
the compiled classes would have to be located in the WEB-INFclassesmurachemail
directory.
Since TextPad is designed for working with Java, you can use it to compile
regular Java classes. However, you may need to configure your system as
described in appendix A before it will work properly. In particular, you
may need to add the appropriate WEB-INFclasses directory to your classpath.
If you use the DOS prompt window to compile your classes, you can do that
as shown in this figure. Here, a DOS prompt is used to compile the User
class. To start, the cd command changes the current directory to the WEB-INFclasses
directory. Then, the javac command is used with "businessUser.java"
as the filename. This compiles the User class and stores it in the
business package, which is what you want.
A JSP that uses the User and UserIO classes
Figure 4-11 shows the code for the JSP in the Email List application after
it has been enhanced so it uses the User and UserIO classes to process the
parameters that have been passed to it. In the first statement of the
body, a special type of JSP tag is used to import the business and data
packages that contain the User and UserIO classes. You'll learn how to
code this type of tag in the next figure. For now, though, you should
focus on the other shaded lines in this JSP.
Figure 4-11: A JSP that uses the User and UserIO classes
The code for a JSP that uses the User and UserIO classes
<!doctype html public "-//W3C//DTD HTML 4.0
Transitional//EN">
<html>
<head>
<title>Chapter 4 - Email List application</title>
</head>
<body>
<%@ page import="business.*, data.*" %>
<%
String firstName = request.getParameter("firstName");
String lastName = request.getParameter("lastName");
String emailAddress = request.getParameter("email");
User user = new User(firstName, lastName, email);
UserIO.addRecord(user, "../webapps/murach/WEB-INF/
etc/UserEmail.txt");
%>
<h1>Thanks for joining our email list</h1>
<p>Here is the information that you entered:</p>
<table cellspacing="5" cellpadding="5" border="1">
<tr>
<td align="right">First name:</td>
<td><%= user.getFirstName() %></td>
</tr>
<tr>
<td align="right">Last name:</td>
<td><%= user.getLastName() %></td>
</tr>
<tr>
<td align="right">Email address:</td>
<td><%= user.getEmailAddress() %></td>
</tr>
</table>
<p>To enter another email address, click on the Back <br>
button in your browser or the Return button shown <br>
below.</p>
<form action="join_email_list.html" method="post">
<input type="submit" value="Return">
</form>
</body>
</html>
Description
-
This
JSP uses a scriptlet to create a User object and add it to a file, and
it uses JSP expressions to display the values of the User object's
instance variables.
-
Since
the User and UserIO classes are stored in the business and data
packages, the JSP must import these packages.
In
the scriptlet of the JSP, the getParameter method is used to get the
values of the three parameters that are passed to it, and these values are
stored in String objects. Then, the next statement uses these strings as
arguments for the constructor of the User class. This creates a User
object that contains the three values. Last, this scriptlet uses the
addRecord method of the UserIO class to add the three values of the User
object to a file named UserEmail.txt that's stored in the WEB-INFetc
directory. Since the WEB-INFetc directory isn't web-accessible, this
prevents users of the application from accessing this file.
After the scriptlet, the code in the JSP defines the layout of the page.
Within the HTML table definitions, the JSP expressions use the get methods
of the User object to display the first name, last name, and email address
values. Although these JSP expressions could use the String objects
instead, the code in this figure is intended to show how the get methods
can be used.
Conclusion
This tutorial is an excerpt from chapter 4 of Murach's Java Servlets and
JSP. If you had any trouble understanding the HTML presented in this
excerpt, or if you had any trouble getting the Email List application to
run under Tomcat, you may want to download chapters 1 through 3 of this
book. Within those chapters, you'll find an HTML tutorial, and you'll find
detailed instructions on how to install and configure the Tomcat servlet/JSP
container.
On the other hand, if you want to learn more about working with JSPs, you
may want to download chapter 4 in its entirety. The remainder of the
chapter shows how to use three more types of JSP tags and how to work with
JSP errors. And if you want to know more about servlets, you may want to
download chapter 5 of this book. This chapter shows how to implement the
Email List application using servlets. Once you learn how JSPs and
servlets work separately, you can learn how to use the best features of
each by using them together within the MVC pattern.
|